Wave Survival Documentation
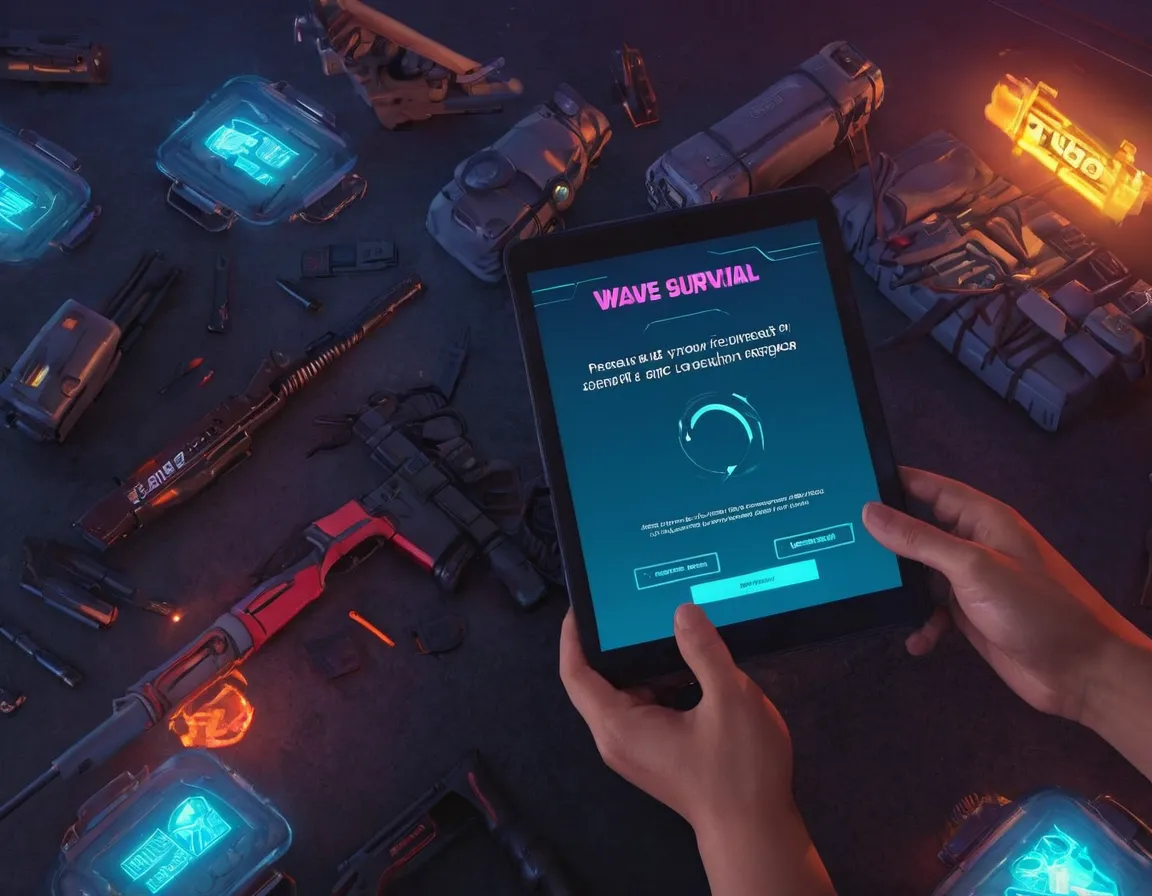
Complete Documentation for Wave Survival
Getting Started
1. Open the Level
To begin using the Wave Survival system, open the level where you want to implement the game.
2. Set Up a Nav-Mesh Bound Volume
A Nav-Mesh Bound Volume is essential for pathfinding and AI navigation within the level. Follow these steps to set it up:
- Go to the Place Actor panel: Open the Place Actor panel in the Unreal Engine Editor.
- Add Nav Mesh Volume: Search for “Nav Mesh Bounds Volume” in the Place Actor panel and drag it into your level. This volume defines the area where the AI can navigate.
After placing the Nav Mesh Bounds Volume in your level, ensure that the area is covered properly by adjusting its size. You can do this by selecting the volume and scaling it to encompass all areas where you want AI movement to occur.
3. Set Up BP_GameMode_Default as the Game Mode
For the game to work with the desired mechanics and logic, you need to assign the correct Game Mode:
- Open the World Settings panel: In the main editor window, go to the World Settings panel.
- Assign BP_GameMode_Default: Under the Game Mode section in the World Settings, set the GameMode to
BP_GameMode_Default
. This blueprint contains the logic for managing the game state, including wave spawns and victory conditions.
4. Set Up the Wave System
The wave system manages the spawning of enemies and controls the progression of waves in the game. Follow the “How to Set Up Wave Spawning” steps to set it up.
How to Set Up Wave Spawning
1. Place BP_WaveManager Blueprint into the Level
The BP_WaveManager
blueprint controls the spawning of waves and manages their behavior. To set it up in your level:
- Go to the Place Actor panel: Open the Place Actor panel in the Unreal Engine Editor.
- Search for BP_WaveManager: Locate the
BP_WaveManager
blueprint in the content browser. - Drag and Drop into the Level: Drag the
BP_WaveManager
blueprint into the level where you want the wave spawning to occur.
The BP_WaveManager
will serve as the central point for managing and controlling the waves.
2. Add Target Points
Target points are designated spawn locations where enemies will appear. To set up the target points:
- Go to the Place Actor panel: Open the Place Actor panel in the Unreal Engine Editor.
- Add Target Points: Search for “Target Point” and drag it into the level. These will act as spawn locations for the enemies in each wave.
- Position the Target Points: Place the target points in locations where enemies will spawn. Ensure that they are placed in open spaces where there are no other actors to avoid collisions during spawning.
Important: Ensure that the target points are spread out enough so that the spawned enemies have room to move without colliding with other actors or obstacles in the level.
3. Add Target Points to the WaveManager
To link the target points to the BP_WaveManager
for use in the wave system, follow these steps:
- Select BP_WaveManager Blueprint: Click on the
BP_WaveManager
blueprint in your level to select it. - Access the Target Point List Variable: In the details panel, locate the
Target Point List
variable within theBP_WaveManager
blueprint. - Add Target Points: Add the target points you placed in the level to the
Target Point List
variable by dragging each target point into the list.
This will allow the BP_WaveManager
to use these target points when spawning enemies during the waves.
How to Add a New Pickup
1. Create a New Child Blueprint of _BP_Pickup_Base
Class (or _BP_Weapon_Base
for Weapons)
To add a new pickup to your game, you need to create a new child blueprint based on the base class for pickups or weapons.
- Navigate to the Content Browser: In Unreal Engine, go to the Content Browser.
- Create a New Child Blueprint:
- Right-click and select Blueprint Class.
- In the parent class selection, choose
_BP_Pickup_Base
if it’s a general pickup or_BP_Weapon_Base
if it’s a weapon-specific pickup. - Name the new blueprint based on the type of pickup you want to create (e.g.,
BP_Medkit_Pickup
,BP_Sword_Pickup
).
2. Add Components for Representing the Pickup Class
Once the blueprint is created, you need to add components that visually represent the pickup in the game world.
- Add Static Mesh or Skeletal Mesh:
- Open the newly created blueprint.
- In the Components tab, add a Static Mesh Component (for non-animated pickups like health packs) or a Skeletal Mesh Component (for animated or weapon pickups).
- Select the appropriate mesh for your pickup item (e.g., a medkit model or a sword model).
- Position the Mesh: Adjust the mesh’s position and scale so it aligns properly within the world.
3. Override the OnPickup Function
The OnPickup
function is called when the player interacts with the pickup. Override this function to define the specific behavior of the pickup (e.g., restoring health or giving the player a weapon).
- Override OnPickup:
- In the blueprint, locate the
OnPickup
function. - Right-click within the blueprint’s Event Graph and select Override Function to override
OnPickup
. - Add the specific code for the behavior of your pickup item. For example, for a health pickup, you might add code to restore the player’s health.
- In the blueprint, locate the
4. Add the New Pickup Class to BP_Gamemode_Default
To ensure your new pickup is randomly spawned in the level, you need to add it to the PickupList
in the BP_Gamemode_Default
blueprint.
- Open
BP_Gamemode_Default
: Locate and open theBP_Gamemode_Default
blueprint. - Find the PickupList Variable: In the blueprint, locate the
PickupList
variable, which holds the list of possible pickups that can spawn in the game. - Add Your New Pickup: Add the newly created pickup class (e.g.,
BP_Medkit_Pickup
) to thePickupList
array. - Save the Blueprint: Make sure to save the
BP_Gamemode_Default
blueprint.
Once added to the PickupList
, the new pickup will be eligible to spawn randomly in the level based on the spawning system in the game.
How to Add a New Weapon
1. Create a New Child Blueprint of _BP_Weapon_Base
Class
To add a new weapon to your game, you need to create a new child class of the base weapon class.
- Navigate to the Content Browser: Open the Content Browser in Unreal Engine.
- Create a New Child Blueprint:
- Right-click and select Blueprint Class.
- In the parent class selection, choose
_BP_Weapon_Base
. - Name your new blueprint based on the weapon type (e.g.,
BP_Sword_Weapon
,BP_Rifle_Weapon
, etc.).
2. Set Weapon Information in the Weapon Information Variable
After creating the weapon blueprint, you’ll need to configure its specific attributes, such as damage, ammo count, etc. This is done by setting values in the Weapon Information
variable.
- Open the New Weapon Blueprint: Open the newly created weapon blueprint.
- Locate the Weapon Information Variable: Find the
Weapon Information
variable, which stores the details of the weapon (damage, fire rate, ammo, etc.). - Set Weapon Properties:
- Set the various properties of the weapon (e.g., damage, rate of fire, clip size, reload speed, etc.) in the
Weapon Information
variable. - You can customize these values based on the weapon’s design and desired gameplay effect.
- Set the various properties of the weapon (e.g., damage, rate of fire, clip size, reload speed, etc.) in the
3. Set Up a Pickup Class for the Weapon
To allow the player to pick up the weapon in the game, you need to create a pickup class associated with the weapon.
Create a New Pickup Blueprint:
- Follow the same process as described in the How to Add a New Pickup documentation. Create a new blueprint that is a child of the
BP_Pickup_Base
class. - Inside the pickup blueprint, add the
BP_Weapon_Base
as the item to be picked up when the player interacts with it.
- Follow the same process as described in the How to Add a New Pickup documentation. Create a new blueprint that is a child of the
Link Weapon to Pickup Class:
- In the pickup blueprint, use the
Weapon Information
to specify which weapon should be picked up. - You may also include additional components, such as static meshes or particle effects, to visually represent the weapon pickup in the game world.
- In the pickup blueprint, use the
Ensure Weapon Pickup Logic: Implement any necessary logic in the pickup blueprint (e.g., adding the weapon to the player’s inventory, switching to the weapon, or removing the weapon from the world after pickup).
How to Add More AI Enemies
1. Create a New Child Class of _BP_AI_Enemy_Base
Class
To add a new AI enemy, you need to create a new child blueprint based on the base AI enemy class.
- Navigate to the Content Browser: Open the Content Browser in Unreal Engine.
- Create a New Child Blueprint:
- Right-click and select Blueprint Class.
- In the parent class selection, choose
_BP_AI_Enemy_Base
. - Name the new blueprint based on the type of enemy you want to create (e.g.,
BP_Zombie_Enemy
,BP_Skeleton_Enemy
, etc.).
2. Set Mesh and Animations
After creating the enemy blueprint, configure its visual appearance and animations.
- Open the New Enemy Blueprint: Open the newly created enemy blueprint.
- Set Mesh:
- Add a Static Mesh Component or Skeletal Mesh Component, depending on whether your enemy is static or animated.
- Select the appropriate mesh for your enemy (e.g., a zombie model, robot, or other enemy types).
- Set Animations:
- If your enemy uses animations, assign an Anim Blueprint to the Skeletal Mesh component.
- Choose or create an animation blueprint that controls the enemy’s movement, idle, and attack animations.
3. Modify the Spawn Enemy Function in BP_WaveManager
To make the newly created enemy spawn during waves, modify the spawn logic in BP_WaveManager
.
- Open
BP_WaveManager
Blueprint: Locate and open theBP_WaveManager
blueprint. - Modify Select Node: In the Select node (which determines which enemy type to spawn), add the new enemy blueprint to the list of selectable options.
- Modify Random Integer Node: Adjust the Max Integer value of the Random Integer node to include the new enemy type in the selection process. This ensures that the newly added enemy can spawn randomly in the wave.
By following these steps, you can add new enemies, pickups, and weapons to your Wave Survival game and customize your gameplay experience.
Tags
ocean interaction, unofficial documentation, unreal engine, c++, wave survival documentation, Wave Survival game development, Unreal Engine wave system, Adding AI enemies in games, Game pickups setup tutorial, Creating custom weapons in Unreal Engine, Wave spawning blueprint, Top-down survival game guide, Unreal Engine game design, AI enemy customization in games, Game development tutorial for beginners, Enhancing wave-based games, Weapon system setup Unreal Engine, Blueprint setup for survival games, Unreal Engine pickup spawning, Wave manager blueprint tutorial